In this Article We are going to learn java Tutorials
Java Tutorial Table of contents
• Introduction
• Basic Java Concepts
• Object-Oriented Programming
• Exception Handling
• Java Collections Framework
• File Handling in Java
• Conclusion
Java Tutorial Introduction
Welcome to the world of Java! If you’re new to programming, Java is a great place to start. But what is Java exactly? Simply put, it’s a programming language used to develop software applications. Java has a rich history dating back to the mid-1990s and has become an essential tool for developers worldwide. But why learn Java? For one, it’s versatile and can be used to develop a wide range of applications, from mobile to web-based. Plus, with its robust libraries and frameworks, Java makes developing in-demand software skills more accessible.
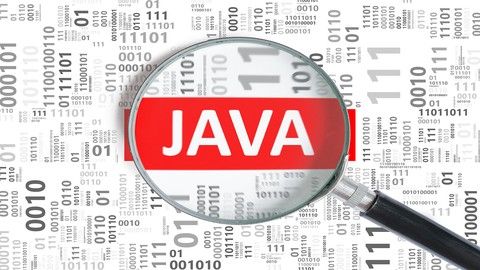
Basic Java Concepts
Alright, alright, alright. Let’s get into the basics of Java, shall we? First things first, let’s talk about variables and data types. A variable is like a labeled box where you can store information. Just like you have different types of boxes for different items, you have different data types to store different types of information – for example, you have int for integers and String for text. Moving on, let’s talk about operators and expressions. Operators are like little helpers that perform operations on variables and values. For example, the + operator is used for addition and the – operator is used for subtraction. Expressions, on the other hand, are combinations of values, variables, and operators that are evaluated to produce a value. Now, let’s dive into conditional statements. These are like forked roads – they take decisions based on certain conditions. For example, if it’s raining outside, you’ll take an umbrella, otherwise, you won’t. In programming, we use if-else statements to make decisions like these. Finally, let’s talk about loops. Loops are used to repeat a section of code multiple times. Think of it like a washing machine – it washes the clothes repeatedly until it’s done. Similarly, a loop executes the same block of code repeatedly until a condition is met. Phew! That was a lot of information to digest, but don’t worry, we’ll revisit these concepts again in the coming sections. For now, pat yourself on the back for making it this far and let’s move on to the next topic.
Table of contents
• Introduction
• Features of Java
• How Does Java Work?
• Java Syntax Basics
• Java Essentials
• Java Tools
• Conclusion
What Is Java: A Comprehensive Guide
Welcome to the world of Java – an island of codes, surrounded by a sea of programs! Java, as you know, is a high-level programming language used for developing applications and software. But do you know how it all started? 23 years ago, Java was designed by James Gosling at Sun Microsystems. It was initially called Oak, but since there was already a programming language of that name, they renamed it Java. Java was created to provide a user-friendly platform that could run on different operating systems. Java has become increasingly popular among developers due to its platform independence, meaning it can run on any platform or operating system that supports Java. This is one of the reasons for its widespread use in a variety of applications. Now that you know the history of Java, let’s consider its importance. Java is a vital contributor to the software industry because of its portability, security, efficiency, and multiple functionalities. Its applications are widely used in e-commerce, software, finance, and gaming industries. Let’s dive deeper into the world of Java and explore its features, syntax, and tools.
Features of Java
So you want to know about the FEATURES of Java? Guess what, we love Java for its amazing features! Here are some of our favorites: Platform Independence: Java is the only platform-independent language out there, which means that you can write Java code on one platform (say, Windows) and run it on another (Mac or Linux) without any modifications at all. How cool is that? Object Orientation: Java is an object-oriented language, which means it’s designed to represent real-life objects and concepts in your code. This makes it intuitive and easy to learn, and also makes for cleaner, more modular code. Security: Java has a reputation for being a highly secure language, thanks to the way it handles memory management, garbage collection, and other key security features. This makes it the language of choice for building secure, enterprise-grade apps. There you have it – some of the best features that make Java an incredible language to work with. Now, let’s dive into how Java works under the hood.
How Does Java Work?
Does Java work like magic? Not quite, but it’s still pretty impressive. Java is a compiled language, meaning that code is written in human-readable format and then compiled into bytecode, which is essentially a set of binary instructions for the Java Virtual Machine (JVM) to execute. This bytecode is then interpreted by the JVM at runtime, which is where Java’s platform independence comes into play. Because the bytecode is essentially a set of instructions for the JVM, it doesn’t matter what type of system you’re running Java on – as long as the JVM is compatible, your Java code should run smoothly. Of course, there’s a little more to it than that. Java’s extensive libraries and support for object-oriented programming make it a powerful language for building complex applications. And with tools like Eclipse and NetBeans, Java development is more accessible than ever. But at its core, Java’s ability to compile into bytecode and run on any system with a compatible JVM is what makes it truly remarkable. Just don’t forget to thank the JVM for all its hard work.
Java Syntax Basics
Java is known for its simplicity, reliability and platform independence. Among the essential aspects of the language, there are four which stand out: Data Types, Variables, Operators and Control Statements. In Java, data types categorise variables and constants, which declare the data’s values that it can store. These types are divided into two categories: primitive data types such as int, boolean, and char, and non-primitive data types such as arrays, classes, and interfaces. Variables in Java are containers that store data values assigned to it. Each variable must be assigned a specific data type during declaration, and its value can change during the program’s execution. Operators can be arithmetic, relational or logical. Arithmetic operators include addition (+), subtraction (-), multiplication (*), and division (/), while relational operators are used to compare two values. Logical operators, such as AND (&&) and OR (||), are used to combine multiple conditions. Control statements are essential in Java programming as they execute specific instructions based on the conditions given. If and else statements are commonly used control statements, with decision-making statements providing an overview of what code to execute when a condition exists. Java is versatile and can handle simple and complex programming activities, making it one of the most popular and widely used programming languages globally.
Java Essentials
Java Essentials: Arrays, Inheritance, Interface, and Exception Handling Arrays are essential in Java as they allow programmers to store a collection of similar type of elements. Arrays can be of a primitive data type or an object. Accessing an element in an array involves referencing the element’s index number. Arrays in Java are fixed in size and cannot be resized during runtime. Inheritance in Java allows a class to inherit properties and behaviour of another class. This concept promotes code reusability and allows for more efficient code development. Subclasses can change or add functionality by overriding or extending the methods of the superclass. Interfaces provide a way for Java to achieve abstraction. An interface specifies a set of methods that a class must implement. Multiple inheritance is possible through interfaces. Exception Handling is vital in Java programming as it enables the control flow of the program to handle runtime errors gracefully. Exceptions can occur during runtime, and Java has mechanisms to handle and process them seamlessly. Java Essentials – check, and we’re done. Now that we have a basic handle on Java, let’s dive into the cool tools that make programming in Java easy!
Java Tools
When it comes to Java Tools, two options that come to mind are Eclipse and NetBeans. Eclipse is a widely-used open-source platform that offers a comprehensive set of tools for Java development. NetBeans, on the other hand, is another popular integrated development environment that provides robust features for Java programming. Both options have their pros and cons, so it’s important to choose the one that better suits your requirements. When it comes to personal preferences, it all boils down to your own tastes, so give them both a try and see which one you prefer!
Object-Oriented Programming
So, we’ve talked about the basics of Java, but let’s get into the meaty stuff: object-oriented programming. This paradigm is commonly used in programming, and Java really shines here. Basically, object-oriented programming is all about building classes and objects that can be used to model real-world objects. A class is like a blueprint that defines the common attributes and methods of a specific type of object. In Java, you can think of a class as a template that you can use to create individual objects. For example, you could create a class called “Person” that defines attributes like name, age, and gender. Once you’ve created a class, you can then use it to create objects that have specific values. So, if you created a “Person” class, you could then create an object called “Bob” with the name “Bob,” the age “42,” and the gender “Male.” Inheritance is another important concept in object-oriented programming. This allows you to create new classes based on existing ones, essentially inheriting their properties and methods. So, if you had a class called “Animal” with properties like “breathing” and “eating,” you could create a new class called “Cat” that inherits those properties, but also has its own unique methods and properties. Polymorphism is a fancy term that basically means “many shapes.” In Java, this refers to the ability to use the same interface for different types of classes. So, if you had two classes called “Dog” and “Cat” that both implemented the “Animal” interface, you could use the same code to interact with both types of animals. Abstraction and encapsulation are two more concepts that are essential to object-oriented programming. Abstraction refers to the ability to hide certain details and only show the relevant information. For example, a class might expose a method that calculates a certain value, but hide the underlying algorithm used to perform the calculation. Encapsulation is all about keeping data and methods separate and organized. This makes it easier to manage code and avoid conflicts between different parts of the program. Overall, object-oriented programming is a powerful tool for building complex programs with clear organization and structure. With Java, you have access to a wide range of tools and concepts that can help you create robust and scalable code.
Exception Handling
Exception Handling is an essential concept in Java programming. It deals with unforeseen errors that may occur while running Java programs. These “exceptions” can be of various types, from simple errors like dividing by zero to complex issues like invalid input data. Java has various types of exceptions that are categorised into two: checked exceptions and unchecked exceptions. Checked exceptions must be dealt with by the programmer, while unchecked exceptions do not require any special handling. The try-catch block is a construct used to handle exceptions in Java. It tries to execute the code and catches any exception that may occur. With the try-catch block, the programmer can control what happens when an exception occurs, and avoid an abrupt termination of the program. Overall, Exception Handling ensures the robustness of Java programs, and we must follow best practices to avoid unnecessary errors.
Java Collections Framework
Let’s talk about the Java Collections Framework, because let’s face it, what is programming without a little bit of collecting? The Java Collections Framework consists of five different collection interfaces, including List, Set, Queue, Deque, and Map. The most commonly used collection types are ArrayList, LinkedList, HashMap, HashSet, and HashTable. ArrayList is great when you need fast access to elements in a list, while LinkedList is your go-to when you need fast insertion and deletion of elements. HashMap is key-value based and is used when you need to store and retrieve elements quickly, and HashSet stores a set of unique elements. Lastly, HashTable is similar to HashMap, but it is synchronized and therefore thread-safe. Overall, knowing and understanding the different types of collections is crucial when it comes to writing efficient and effective Java code. So, pick your collection type wisely and watch your code thrive!
File Handling in Java
Now, let’s talk about file handling in Java. Reading and writing files is an important aspect of any programming language. Java provides a set of APIs for creating, processing and analyzing files. Reading and writing files in Java is pretty straightforward. With just a few lines of codes, you can easily perform these operations. Working with directories is also a piece of cake in Java. With the java.nio.file package, you can create, move, delete and list directories and their contents effortlessly. Similarly, handling streams is essential in file processing. Java provides a wide range of stream classes such as FileInputStream, FileOutputStream, DataInputStream, etc., to perform these tasks. With streams, you can perform various operations on data such as reading, writing, copying, and many more. In a nutshell, file handling is an integral part of Java programming, and it is vital to understand the basics of it to become a proficient Java developer.
Conclusion
So, what have we learned during our Full Java Tutorial for Beginners? We’ve covered basic Java concepts like variables and data types, and delved into object-oriented programming with classes, objects, inheritance, polymorphism, abstraction, and encapsulation. We’ve also explored exception handling, Java Collections Framework, and file handling in Java. That’s a lot of ground covered! Now, if you want to master Java, make sure to practice, practice, practice. Get comfortable with the syntax and the language constructs, and challenge yourself with more advanced applications. Don’t be afraid to explore different resources and connect with other developers. Because even if you’re a beginner, you can still become a Java pro with determination and hard work. Good luck on your Java journey!
Conclusion
In conclusion, Java is a versatile programming language with platform independence, object-oriented structure, and robust security features. Understanding the language basics, such as data types, variables, operators, and control statements is crucial for writing efficient and error-free code. Additionally, tools like Eclipse and NetBeans make Java programming even more accessible.